Try the Caesar Cipher
Use the tool below to encode or decode a message:
Result:
Wonderful moments in history
Welcome to the world of Caesar Cipher
This realm intertwines simplicity and intrigue in the art of cryptography. Originating from the practices of Julius Caesar, this cipher serves as a testament to the timeless allure of secret communications. It operates on a straightforward yet ingenious principle – shifting the letters of the alphabet by a fixed number. This shift transforms ordinary messages into cryptic texts, cloaking words in a veil of mystery. As we delve into this world, we unravel the elegance of its simplicity and the joy of decoding messages that once seemed impenetrable. The Caesar Cipher, though elementary, opens the gateway to the broader, fascinating world of cryptography, where every letter and shift play a crucial role in the dance of secrecy and discovery.
What is Caesar Cipher?
The Caesar Cipher, an ancient encryption technique, gained fame through Julius Caesar, the renowned Roman general and politician. He used this method to safeguard crucial military communications. Classified as a substitution cipher, it specifically employs an alphabetical shift. The fundamental principle of the Caesar Cipher involves offsetting each letter in the alphabet by a predetermined number. For instance, with an offset of 3, the alphabet shifts such that 'A' becomes 'D', 'B' becomes 'E', and this pattern continues. Upon reaching the end of the alphabet, the sequence circles back to the beginning.
While the Caesar Cipher often serves as a foundational element in more complex encryption methods, its simplicity renders it vulnerable. Like all ciphers based on alphabet substitution, it is relatively easy to decipher, thus offering limited security for practical communication needs.
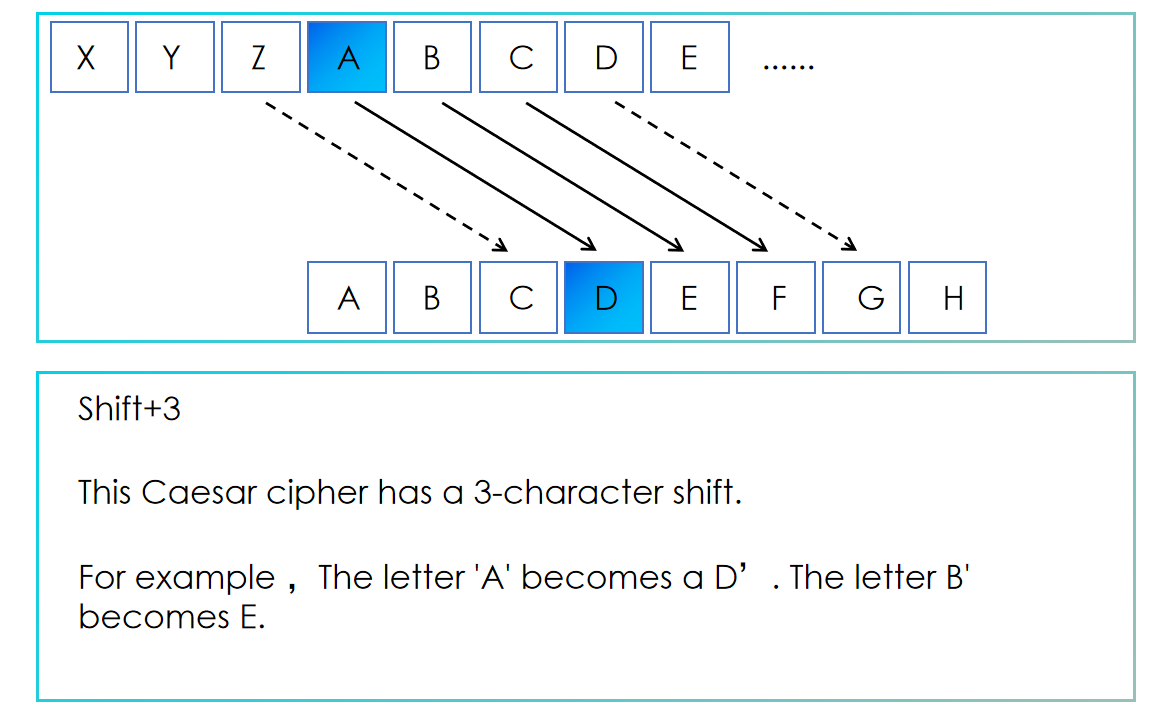
What are the specific Caesar Ciphers?
The Caesar Cipher is a method of encryption that involves shifting the letters of the alphabet by a fixed number either backwards or forwards. The simplest form is to shift each letter by a fixed number. However, besides this basic form, here are some more interesting variants:
- ROT13: A special case of the Caesar Cipher with a shift of 13. Since there are 26 letters in the English alphabet, the same rule applies for both encryption and decryption.
- Atbash Cipher: This is a special case that could be considered a Hebrew Caesar Cipher. It reverses the alphabet, so the first letter becomes the last letter, the second letter becomes the second to last, and so on.
- Vigenère Cipher: Although not strictly a Caesar Cipher, it is developed based on the principle of the Caesar Cipher. It uses a keyword as the shift value for encryption, providing higher security compared to a single letter shift.
- Affine Cipher: Based on the idea of the Caesar Cipher, but introduces multiplication in the encryption process. Each letter's position in the alphabet is first multiplied by a number (coprime with the length of the alphabet) and then added to a shift value, finally taking the modulus of the alphabet length to get the encrypted letter.
- ROT5, ROT18, ROT47: These are variants of ROT13 but are used for encrypting numbers and other characters. ROT5 is used only for numbers, ROT18 combines ROT5 and ROT13, and ROT47 can encrypt most printable characters in the ASCII table.
- Double Caesar Cipher: This is a simple extension of the Caesar Cipher, applying the Caesar Cipher twice, possibly with different shift values, to increase the complexity of the encryption.
These variants and related techniques each have their characteristics, aiming to enhance the security of the encryption method or to meet specific encryption needs.
How to implement Caesar Cipher in Python?
In Python, you can do this by looping through each letter of the original text and then calculating the encrypted version of each letter based on the alphabet and a given offset. This is conveniently achieved using an ASCII code table, for example, by taking the difference between the ASCII value of the letter and the ASCII value of 'a', plus an offset, and then converting the result back to the letter.
Python Code for Caesar Cipher
Below is a Python function that demonstrates how to encrypt and decrypt text using the Caesar Cipher technique. The code includes comments for better understanding and adaptability.
def caesar_cipher_enhanced(text, shift, encrypt=True):
"""
Encrypts or decrypts text using Caesar Cipher.
Parameters:
text (str): The text to encrypt or decrypt.
shift (int): The number of positions to shift the letters by.
encrypt (bool): True for encryption, False for decryption.
Returns:
str: The transformed text.
"""
transformed_text = ""
for char in text:
if char.isalpha():
start = ord('A') if char.isupper() else ord('a')
shift_adjusted = shift if encrypt else -shift
transformed_char = chr((ord(char) - start + shift_adjusted) % 26 + start)
transformed_text += transformed_char
else:
transformed_text += char
return transformed_text
# Example usage
user_input = input("Enter the text: ")
shift = int(input("Enter the shift value: "))
encrypt_decrypt = input("Encrypt or Decrypt (E/D): ").strip().upper()
if encrypt_decrypt == 'E':
result = caesar_cipher_enhanced(user_input, shift, encrypt=True)
print("Encrypted:", result)
elif encrypt_decrypt == 'D':
result = caesar_cipher_enhanced(user_input, shift, encrypt=False)
print("Decrypted:", result)
else:
print("Invalid option. Please enter 'E' for Encrypt or 'D' for Decrypt.")
How to crack the Caesar Cipher?
Cracking a Caesar Cipher can be relatively simple due to the limited number of possible shifts (26 in the case of the English alphabet). A common method to break this cipher is by brute force, which means trying out every possible shift until you find one that makes sense. This is practical because there are only 26 possible shifts in the English alphabet, making the number of combinations small enough to check each one manually.
Another more refined method is to use frequency analysis. Since letters in the English language have different frequencies of occurrence (for example, 'e' is more common than 'z'), you can compare the frequency of letters in the encoded message with typical letter frequencies in English. By doing this, you can identify the most probable shift that was used to encrypt the message.
Since the mapping of each character in the Caesar Cipher is fixed, if "b" maps to "e", then "e" will appear in the ciphertext every time "b" appears in the plaintext. It is now known that the probability distribution of each letter in English is known. The average probability of occurrence of different letters in different texts is usually the same, and the longer the text, the closer the frequency calculation is to the average. This is a frequency chart of 26 letters. Of course, as the number of samples changes, the frequency of each letter will be slightly different.
For example, enter the first paragraph of text "This realm intertwines simplicity and intrigue...", and through the converter above we get the ciphertext. But for others who don't know what the secret key is, we can get a secret key through the code, which is the offset.
The original English text is as follows:
"This realm intertwines simplicity and intrigue in the art of cryptography. Originating from the practices of Julius Caesar, this cipher serves as a testament to the timeless allure of secret communications. It operates on a straightforward yet ingenious principle – shifting the letters of the alphabet by a fixed number. This shift transforms ordinary messages into cryptic texts, cloaking words in a veil of mystery. As we delve into this world, we unravel the elegance of its simplicity and the joy of decoding messages that once seemed impenetrable. The Caesar Cipher, though elementary, opens the gateway to the broader, fascinating world of cryptography, where every letter and shift play a crucial role in the dance of secrecy and discovery."
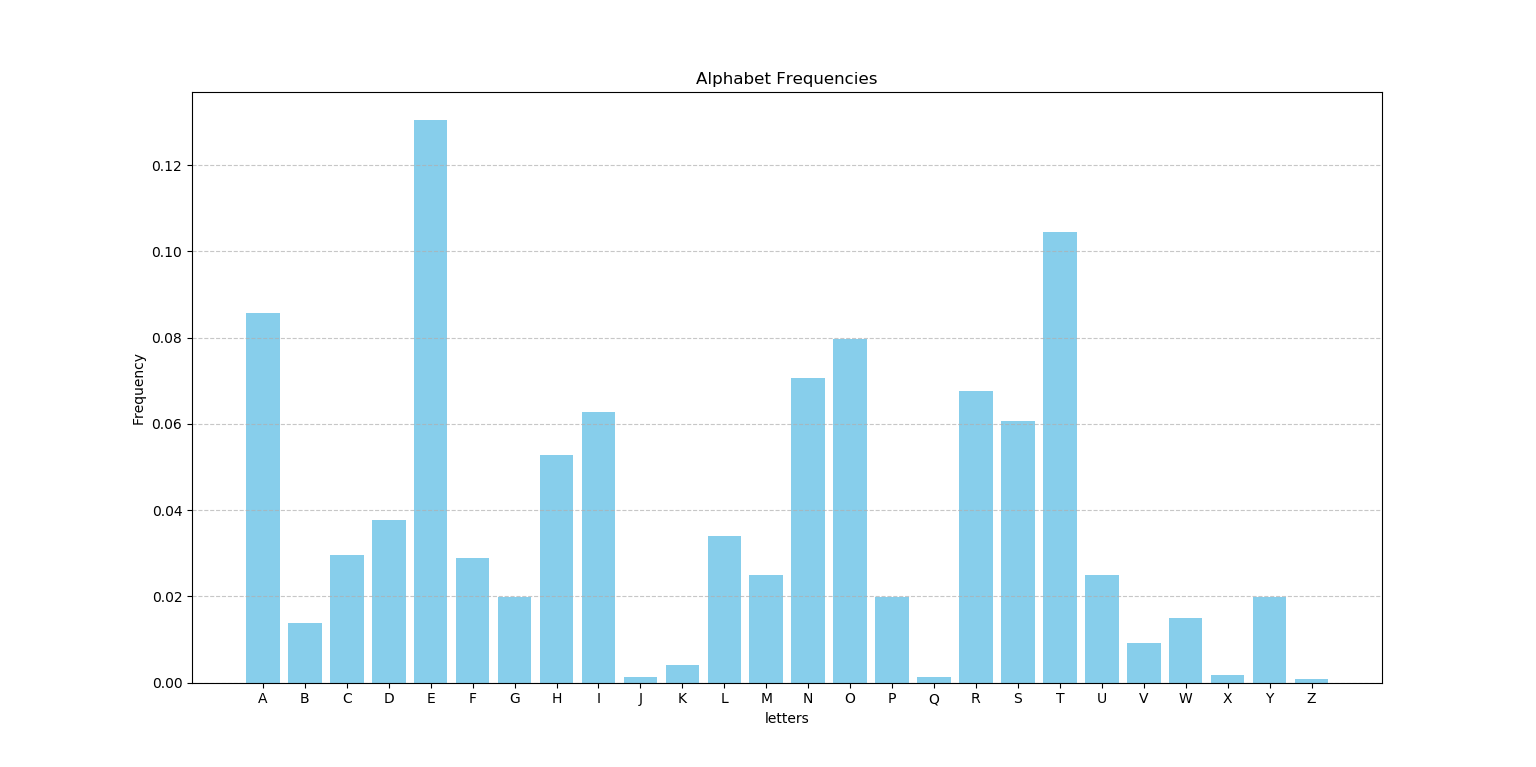
The following Python code example demonstrates how to perform frequency analysis to break a Caesar Cipher. This technique is based on the statistical analysis of letter frequencies in English.
import string
def count_frequencies_from_file(path):
count_dict = dict.fromkeys(string.ascii_lowercase, 0)
total_chars = 0
with open(path, 'r', encoding='utf-8') as file:
for line in file:
for char in line.lower():
if char in count_dict:
count_dict[char] += 1
total_chars += 1
for char in count_dict:
count_dict[char] /= total_chars
return count_dict
def frequency_analysis(known_frequencies, count_dict):
eps = float('inf')
key = 0
cipher_frequencies = list(count_dict.values())
for shift in range(26):
s = 0
for i in range(26):
s += known_frequencies[i] * cipher_frequencies[(i + shift) % 26]
temp = abs(s - 0.065379)
if temp < eps:
eps = temp
key = shift
return key
# Known letter frequencies in English
known_freqs = [0.086,0.014,0.030,0.038,0.130,0.029,0.020,0.053,0.063,0.001,0.004,0.034,0.025,0.071,0.080,0.020,
0.001,0.068,0.061,0.105,0.025,0.009,0.015,0.002,0.020,0.001]
file_path = "Your_Path"
cipher_count_dict = count_frequencies_from_file(file_path)
key = frequency_analysis(known_freqs, cipher_count_dict)
print("The key is: " + str(key))
Try using your own file path and run this code to see if you can decrypt a message encrypted with a Caesar Cipher.
Caesar Cipher and the cipher used by Caesar
In Chapter 56 of Suetonius's De Vita Caesarum, the use of encryption techniques in Caesar's private letters is described:
"Extant et ad Ciceronem, item ad familiares domesticis de rebus, in quibus, si qua occultius perferenda erant, per notas scripsit, id est sic structo litterarum ordine, ut nullum verbum effici posset: quae si qui investigare et persequi velit, quartam elementorum litteram, id est D pro A et perinde reliquas commutet." ( Suetonius, De Vita Caesarum: Divus Iulius. The Latin Library, accessed June 1, 2024, https://www.thelatinlibrary.com/suetonius/suet.caesar.html )
This content does not directly mention the modern concept of the Caesar Cipher, which involves simple letter shifting encryption. Instead, the encryption method used by Caesar was more akin to a transposition cipher, where the actual positions of the letters are changed to encrypt the message, distinctly different from the fixed shift of the Caesar Cipher. This encryption technique involves relatively complex rearrangement and substitution of letters, demonstrating a significant difference from the direct shift method of the modern Caesar Cipher
Steganography and Cryptography
Explore the fascinating work of Johannes Trithemius, a Renaissance-era Benedictine monk and scholar from Germany. He is known for his seminal work, Steganographia, which delves into the realms of steganography and also touches upon cryptographic techniques in his other scholarly works.
Steganographia is often misinterpreted as purely involving magical elements and summoning spirits. However, it cleverly encases a complex cryptographic system within its narratives. Below is an overview of the cryptographic content encrypted across its volumes:
- Volumes One and Two: These volumes focus predominantly on encryption techniques. Initially perceived as guides for summoning spirits, they metaphorically represent intricate methods of cryptography. They describe innovative methods for transmitting hidden messages over long distances, a groundbreaking concept at the time.
- Volume Three: This volume extends some of the earlier themes but introduces more controversial discussions that deviate from the straightforward cryptography found in the first two volumes. It openly explores more spiritual and magical dimensions, which have been subject to varied interpretations through the ages.
Through deeper analysis, Trithemius's work reveals that what appears as magical invocations are actually veiled descriptions of cryptographic methods, highlighting the relevance of cryptography similar to modern cryptographic practices.
Definitions and Basic Principles
Steganography is the art of concealing information within non-sensitive media such as images, audio, or video files, rendering the information invisible to casual observers. The essence of steganography lies in obscuring the existence of the information, not just its content.
Cryptography, in contrast, involves converting plaintext information into a secure encrypted format that cannot be understood without the corresponding decryption key, relying on complex mathematical algorithms like public/private key mechanisms and symmetric encryption algorithms.
Technical Implementation and Applications
The implementation of steganography typically involves encoding secret information into various parts of ordinary files, such as the pixels in images, videos, audio, or even text. This type of information hiding is achieved through subtle modifications, for example, fine-tuning the pixel values in an image, altering the least significant bit (LSB) of color values, or adding signals in audio files that are beyond human hearing frequencies. These modifications are below the threshold of human perception, so even when the information is transmitted or displayed, it remains undetectable to outside observers. The advantage of this method is that even direct visual observation cannot easily discern these subtle changes.
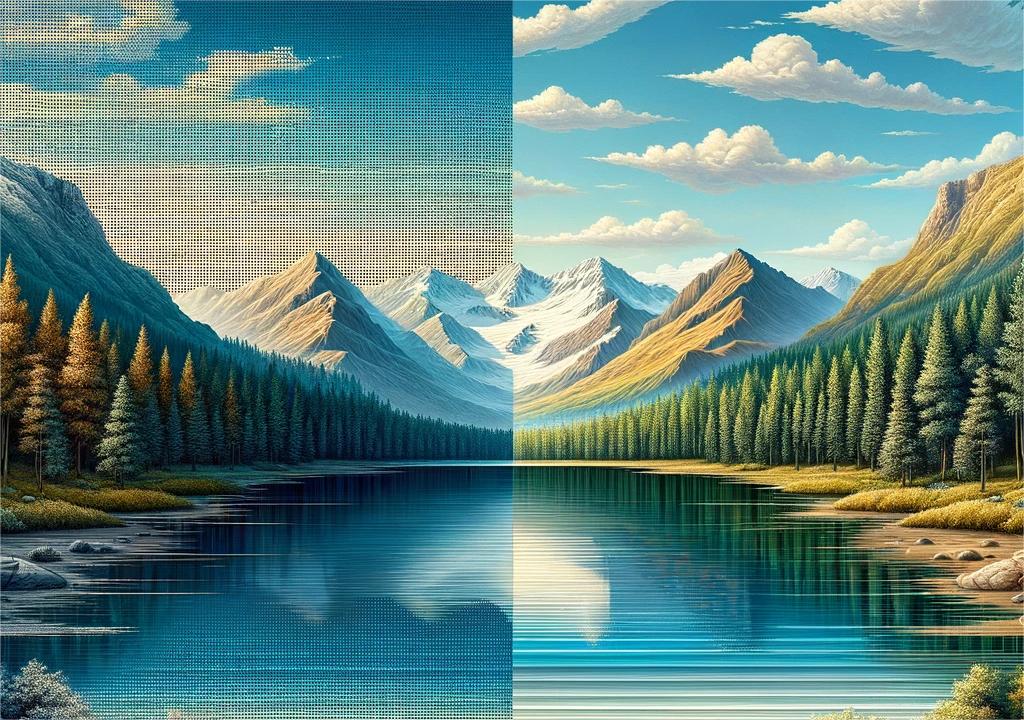
The image above illustrates the concept of steganography. The left side of the image, labeled as 'normal,' shows no alterations and retains the original appearance of the landscape. Conversely, the right side demonstrates how text can be hidden using the LSB method, altering the image subtly enough to make the changes nearly invisible.
Cryptography transforms sensitive information using cryptographic keys to create ciphertext. This encrypted format ensures that data remains secure and unintelligible to unauthorized entities, crucial for protecting communications and data across various systems.
Detection and Security
The security of steganography primarily relies on its concealment. Once the use of steganography is suspected, specialized technical analyses, such as statistical analysis or pattern recognition, can be employed to attempt to uncover hidden information. However, if the steganographic method is well-designed, even experts may find it difficult to detect the concealed information.
The security of encryption technology depends on the strength of the encryption algorithms and the secure management of keys. Modern encryption methods, such as AES and RSA, are designed to withstand various types of attacks, including those from quantum computing. The confidentiality of the keys is critical to encryption security; once the keys are compromised, the encryption protection is broken.
Appropriate Environments and Limitations
Steganography is ideal for highly confidential scenarios, such as covert communications, where revealing the existence of the information could be detrimental. However, it is limited by the volume of data that can be effectively concealed. On the other hand, cryptography is versatile, suitable for securing data in a broad range of applications from financial transactions to personal data protection, though it demands rigorous key management to prevent security breaches.
In summary, both steganography and cryptography play critical roles in the field of information security. They can be employed individually or in tandem to offer robust protection, tailored to specific security needs and the nature of the threats involved.